Tag extra fields and meta data
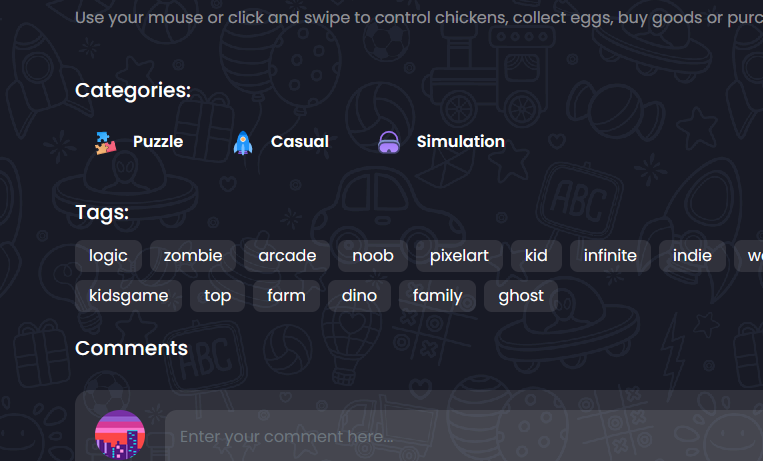
Initially, we build game’s “Tag” feature with basic properties, without description, tag title and more.
As of CloudArcade v1.7.6, the “Extra Fields” plugin has been implemented for the “Tag” feature, allowing you to extend its capabilities.
To utilize extra fields for tags, you’ll need at least version 1.0.3 of the “Extra Fields” plugin and version 1.0.2 of the “Tag Manager” plugin.
Example usage:
Adding a Tag Description:
Navigate to “Plugins > Extra Fields > [ Tag ] Load extra fields > Add new”
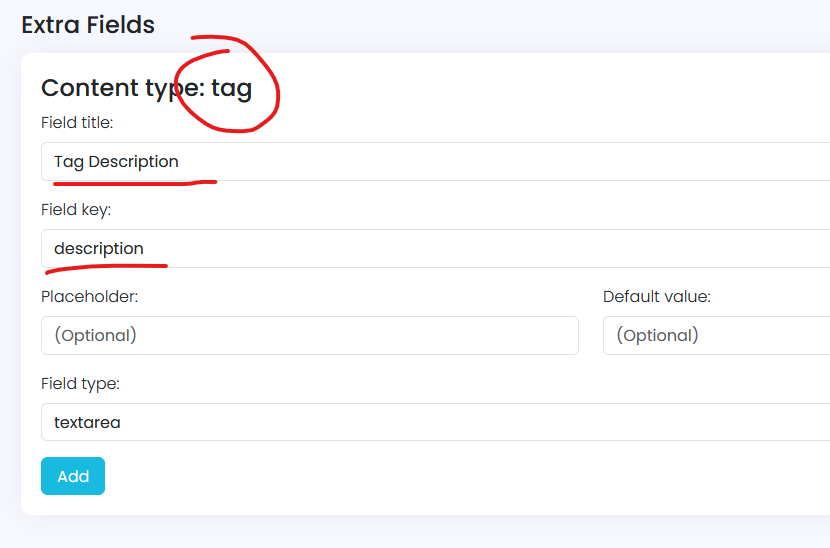
Fill it like image above, then click “Add”
After the new description field successfully added, go to “Plugins > Tag Manager”, then click the edit icon for a tag you want to edit.
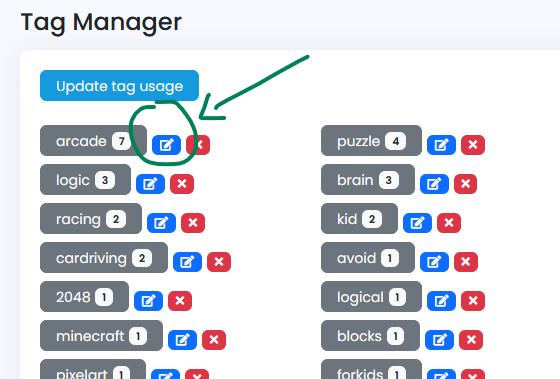
Fill the “description” field, then click “Save”.
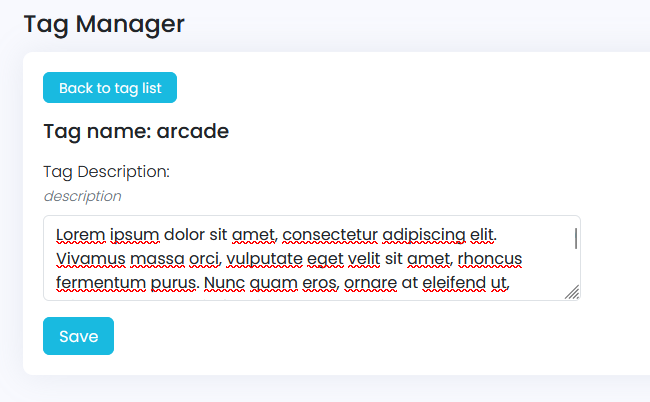
Note, Extra fields are not displayed automatically; you’ll need to manually integrate them into your theme code.
Displaying the Tag Description:
Go to “Plugins > Theme Editor” and select “tag.php”
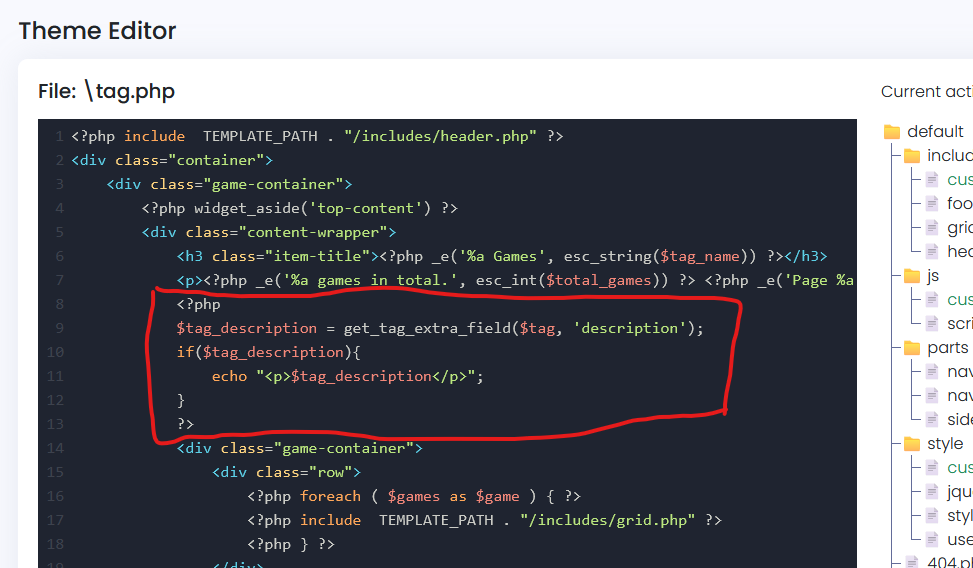
Put the code above <div class=”game-container”>
<?php
$tag_description = get_tag_extra_field($tag, 'description');
if($tag_description){
echo "<p>$tag_description</p>";
}
?>
Here is the result:
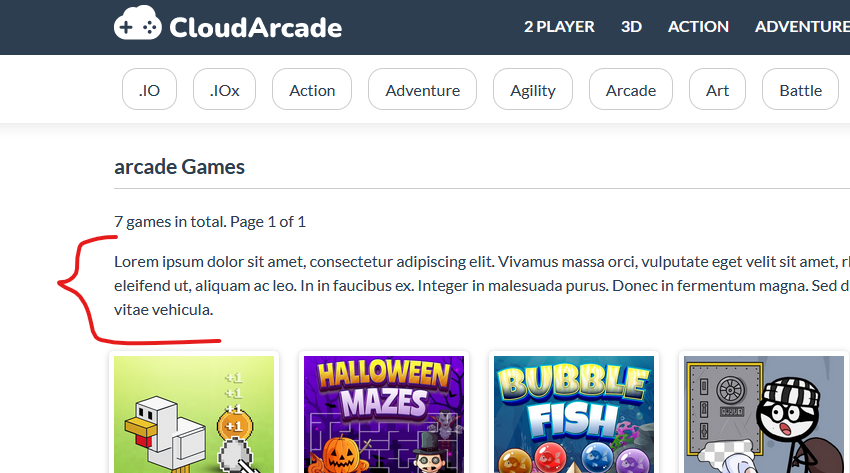
The code above checks if the current tag has a “description” and displays it if available.
Adding Tag Title:
Navigate to “Plugins > Extra Fields > [ Tag ] Load extra fields > Add new”
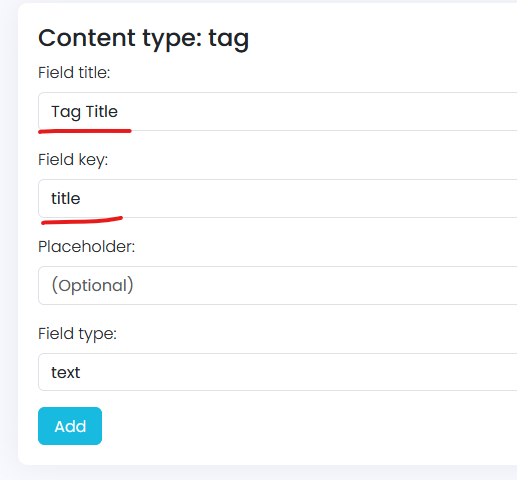
Then click “Add”
Implement Tag Title:
Go to “Plugins > Theme Editor” and select “custom.php”
We will override the default get_page_title()
with our custom page title.
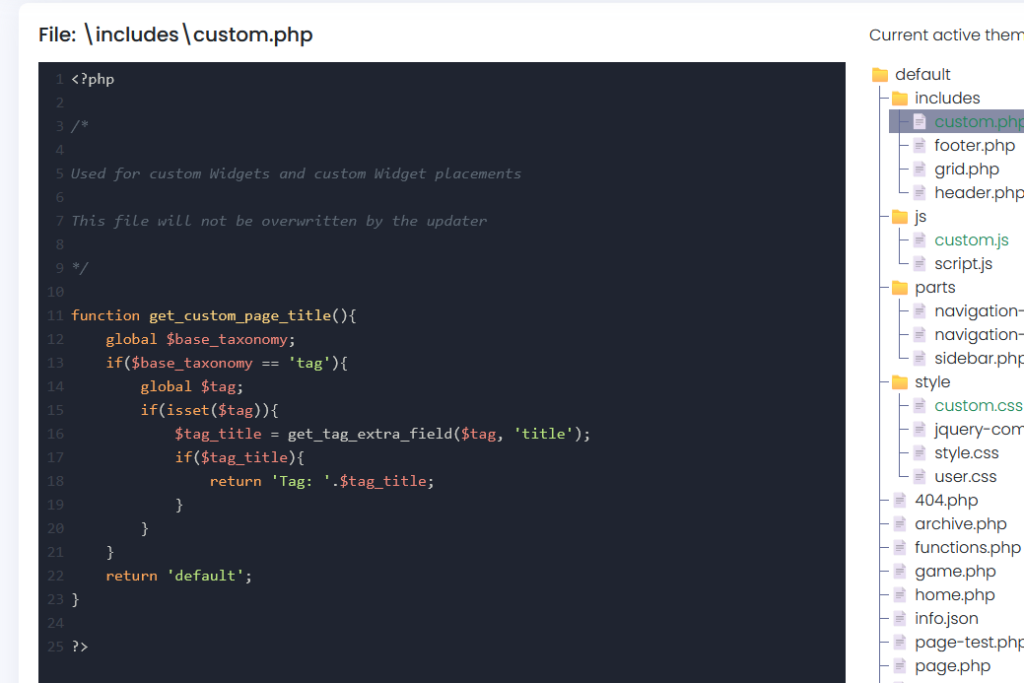
Code:
function get_custom_page_title(){
global $base_taxonomy;
if($base_taxonomy == 'tag'){
global $tag;
if(isset($tag)){
$tag_title = get_tag_extra_field($tag, 'title');
if($tag_title){
return 'Tag: '.$tag_title;
}
}
}
return 'default';
}
Adding Tag Meta Description:
Navigate to “Plugins > Extra Fields > [ Tag ] Load extra fields > Add new”
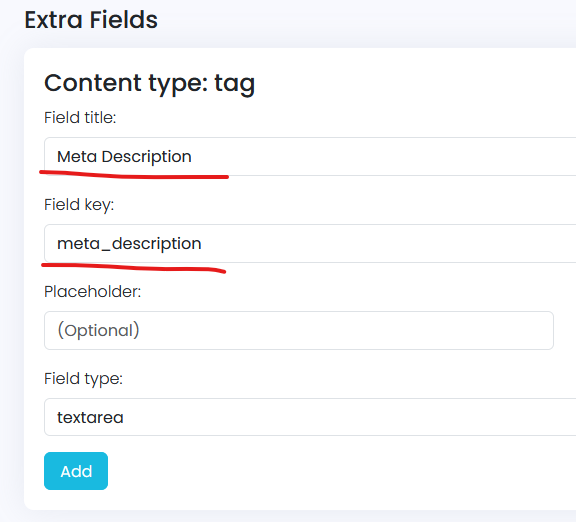
Implement Meta Description:
Go to “Plugins > Theme Editor” and select “custom.php”
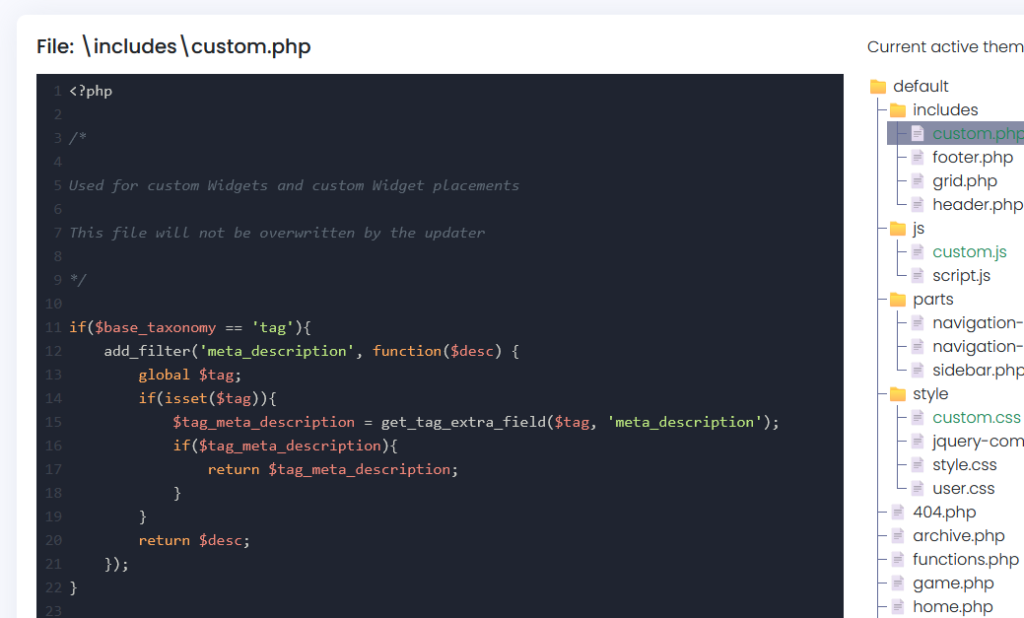
Code:
if($base_taxonomy == 'tag'){
add_filter('meta_description', function($desc) {
global $tag;
if(isset($tag)){
$tag_meta_description = get_tag_extra_field($tag, 'meta_description');
if($tag_meta_description){
return $tag_meta_description;
}
}
return $desc;
});
}
Normally, for other content type, we get the Extra field with this code content->getExtraField('key')
For tag, we use get_tag_extra_field($tag, 'key')
, because Tag is not a Class like other content type.