Create “Load More” games with Custom Page
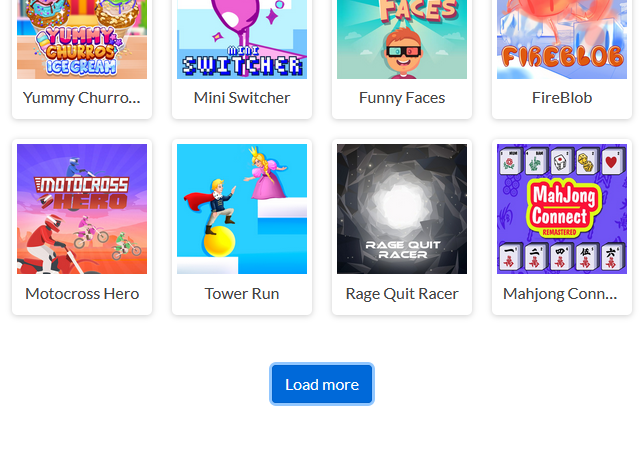
UPDATE: The Default and Dark Grid themes have already implemented the “Load More Games” button in the homepage’s New Games section, starting from theme version v1.1.7.
With “Load more” games button, player or visitor can expand a group of games easily.
Let’s get started how to create it. this tutorial require a coding.
Make sure you’re using CloudArcade v1.2.9 or newer
If you’re not familiar with Custom Page, read this tutorial
First step, open your active theme folder, then create a php file called “page-load-more.php”, you can rename “load-more” with other page name you want later.
Put code below inside “page-load-more.php”
<?php
$page_title = 'Load More';
$meta_description = 'Load more';
require_once( TEMPLATE_PATH . '/functions.php' ); //Load theme functions
include TEMPLATE_PATH . "/includes/header.php";
?>
<ul id="game-list"></ul>
<button id="load-more">Load more</button>
<script type="text/javascript">
var last_offset = 0;
var load_amount = 12;
document.addEventListener("DOMContentLoaded", function(event) {
$('#load-more').click(()=>{
fetch_games(load_amount, 'new');
});
function fetch_games(amount, sort_by) {
$.ajax({
url: "/includes/fetch.php",
type: 'POST',
dataType: 'json',
data: {amount: amount, offset: last_offset, sort_by: sort_by},
complete: function (data) {
append_content(JSON.parse(data.responseText));
}
});
}
function append_content(data){
last_offset += data.length;
data.forEach((item)=>{
$("#game-list").append('<li>'+item['title']+'</li>');
});
if(data.length < load_amount){
$('#load-more').hide();
}
}
})
</script>
<?php
include TEMPLATE_PATH . "/includes/footer.php"
?>
Visit the page ( yourdomain.com/load-more ), It will look like this:
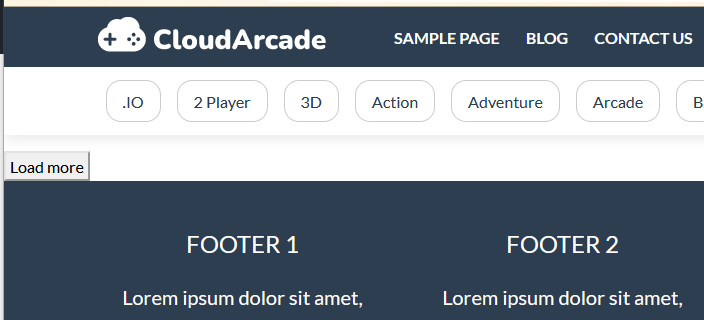
If you click “Load more” button, game list will be shown
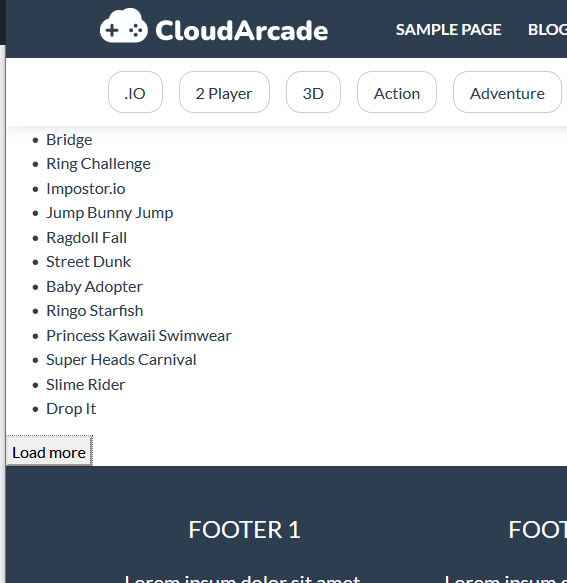
The looks is very basic, we will make it fit with current theme later.
Let me explain the fuctions
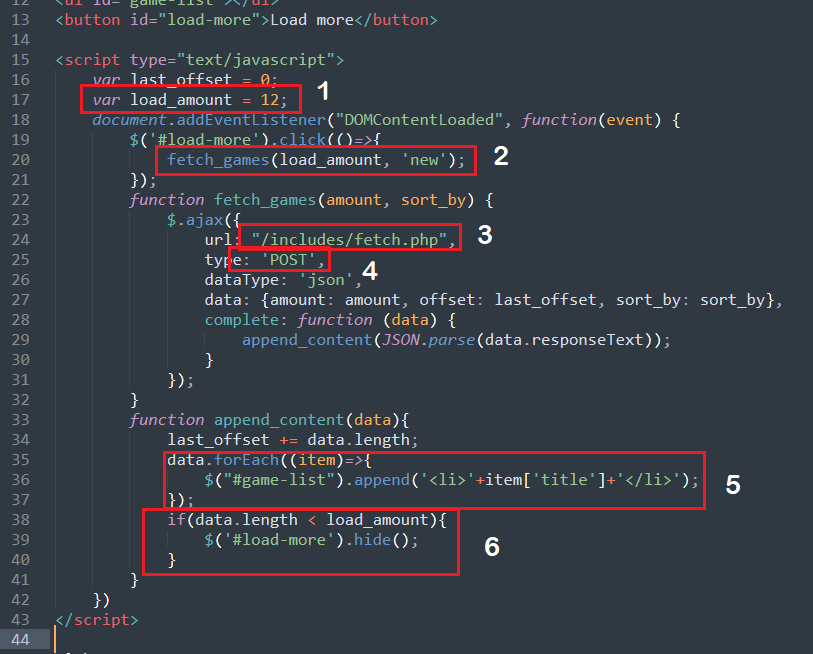
- Number of games you want to load
- Call fetch games function. We want to get 12 games, sort by newest. There also another options ‘new’, ‘random’, ‘popular’ and ‘likes’
- PHP script path to get game list from the database, if you’re installing CloudArcade in a sub-folder, change path to “/your-folder/includes/fetch.php”
- “type” must be “POST” or it will not returning anything
- Get the list or result and append it as HTML
- If there are no more games, remove the “Load more” button
Let’s implement grid for game list to make it match with current theme design
Update all the code from “page-load-more.php” with code below:
<?php
$page_title = 'Load More';
$meta_description = 'Load more';
require_once( TEMPLATE_PATH . '/functions.php' ); //Load theme functions
include TEMPLATE_PATH . "/includes/header.php";
?>
<div class="container">
<div class="game-container">
<div class="row" id="game-list">
<?php
$games = get_game_list('new', 12)['results'];
foreach ( $games as $game ) { ?>
<?php include TEMPLATE_PATH . "/includes/grid.php" ?>
<?php } ?>
</div>
</div>
</div>
<div class="text-center">
<button id="load-more" class="btn btn-primary mb-5">Load more</button>
</div>
<script type="text/javascript">
var last_offset = 12;
var load_amount = 12;
document.addEventListener("DOMContentLoaded", function(event) {
$('#load-more').click(()=>{
fetch_games(load_amount, 'new');
});
function fetch_games(amount, sort_by) {
$.ajax({
url: "/includes/fetch.php",
type: 'POST',
dataType: 'json',
data: {amount: amount, offset: last_offset, sort_by: sort_by},
complete: function (data) {
append_content(JSON.parse(data.responseText));
}
});
}
function append_content(data){
last_offset += data.length;
data.forEach((game)=>{
let html = '<div class="col-md-2 col-sm-3 col-4 item-grid">';
html += '<a href="/game/'+game['slug']+'">';
html += '<div class="list-game">';
html += '<div class="list-thumbnail"><img src="'+game['thumb_2']+'" class="small-thumb" alt="'+game['title']+'"></div>';
html += '<div class="list-content">';
html += '<div class="list-title">'+game['title']+'</div>';
html += '</div></div></a>';
html += '</div>';
$("#game-list").append(html);
});
if(data.length < load_amount){
$('#load-more').hide();
}
}
})
</script>
<?php
include TEMPLATE_PATH . "/includes/footer.php"
?>
This is the preview
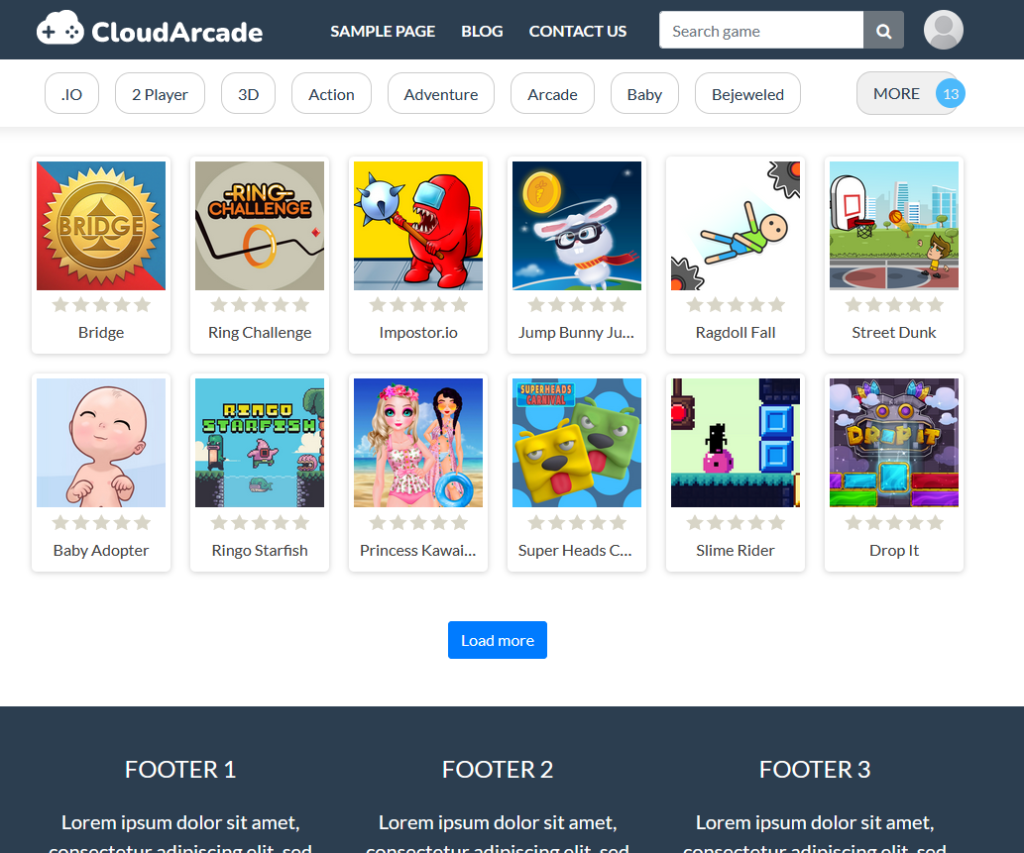
You can put script above on homepage (theme) or anywhere.
You can also fetch games by category
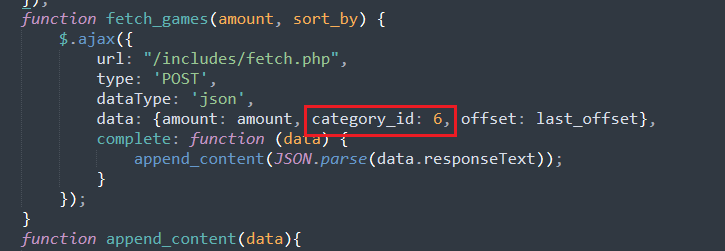
The script difference is, you need to add “category_id”. “sort_by” parameter is not used for Category section, sorted by newest.
Where to get / see category ID ?
Open “Categories” page ( Admin panel ), then click “Edit” icon on a category, you will see category ID.
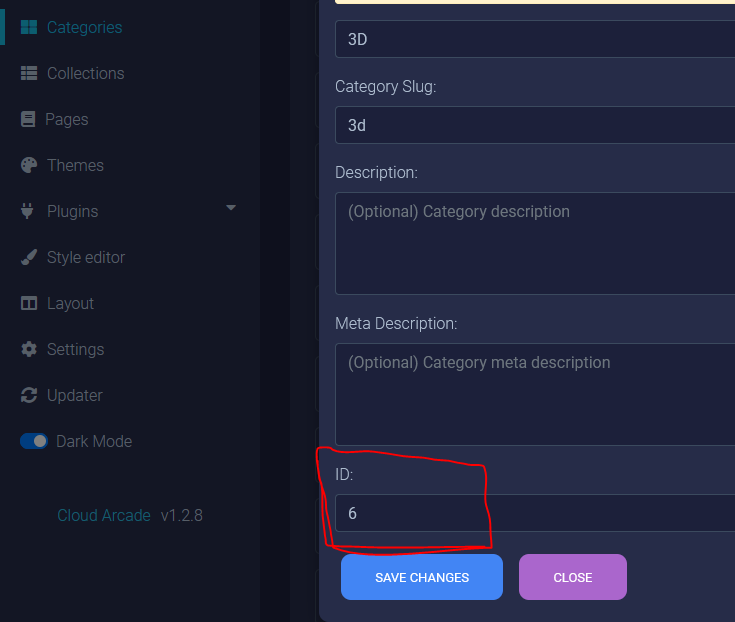