Create a Leaderboard / Scoreboard page with Custom Page
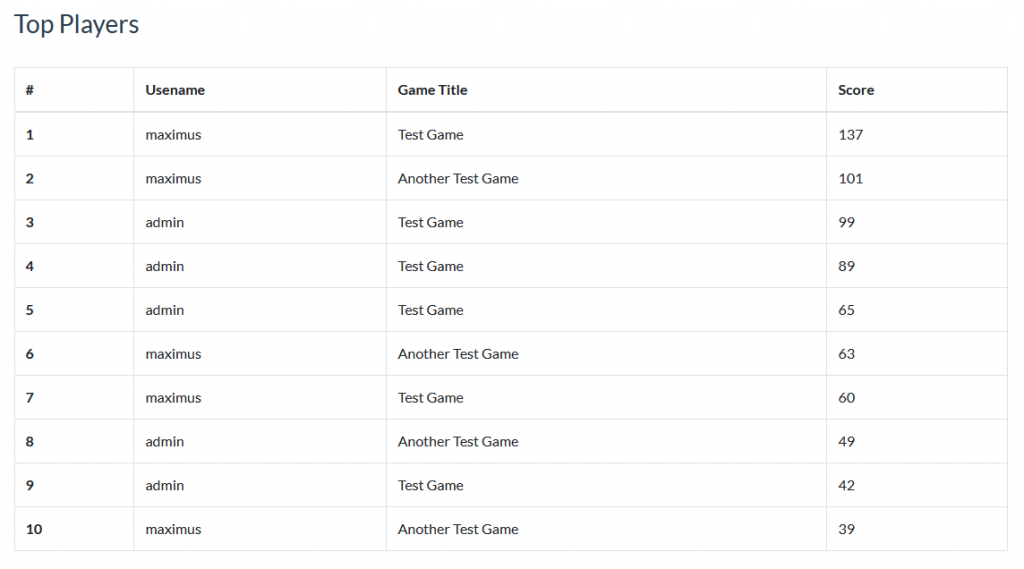
First, open your active theme folder. In this tutorial I will use the default theme “/content/themes/default/”.
Create a php file and name it “page-leaderboard.php”, you can replace “leaderboard” with another page name you want, ex: “page-top-palyers.php”, you can access it with “yout-domain.com/top-players”.
Next, put php code below inside “page-leaderboard.php” file
<?php
$page_title = 'Leaderboard';
$meta_description = 'Top players';
require_once( TEMPLATE_PATH . '/functions.php' ); //Load theme functions
include TEMPLATE_PATH . "/includes/header.php";
// CONTENT
include TEMPLATE_PATH . "/includes/footer.php"
?>
Explain: We include theme functions, header and footer. $page_title and $meta_description is required
Let’s get the recorded scores from database. make sure you’re have a game that already integrated with CloudArcade API and also have submitted score, if not, it will be empty.
Download sample project with API Integration here, then add it with upload game method.
Update “page-leaderboard.php” with code below
<?php
$page_title = 'Leaderboard';
$meta_description = 'Top players';
require_once( TEMPLATE_PATH . '/functions.php' ); //Load theme functions
include TEMPLATE_PATH . "/includes/header.php";
// CONTENT
?>
<div class="container">
<div class="game-container">
<?php
$amount = 10;
$conn = open_connection();
$sql = "SELECT * FROM scores WHERE created_date > DATE_SUB(NOW(), INTERVAL 1 MONTH) ORDER by score DESC LIMIT ".$amount;
$st = $conn->prepare($sql);
$st->execute();
//
$row = $st->fetchAll(PDO::FETCH_ASSOC);
$list = [];
foreach($row as $item){
$game = Game::getById($item['game_id']);
if($game){
$item['game_title'] = $game->title;
$item['username'] = User::getById($item['user_id'])->username;
array_push($list, $item);
}
}
//Show the list
echo '<ul>';
foreach($list as $item){
echo '<li>'.$item['game_title'].', '.$item['username'].', '.$item['score'].'</li>';
}
echo '</ul>';
?>
</div>
</div>
<?php
include TEMPLATE_PATH . "/includes/footer.php"
?>
Preview the result, go to “your-domain.com/leaderboard”.
The result will look like this:
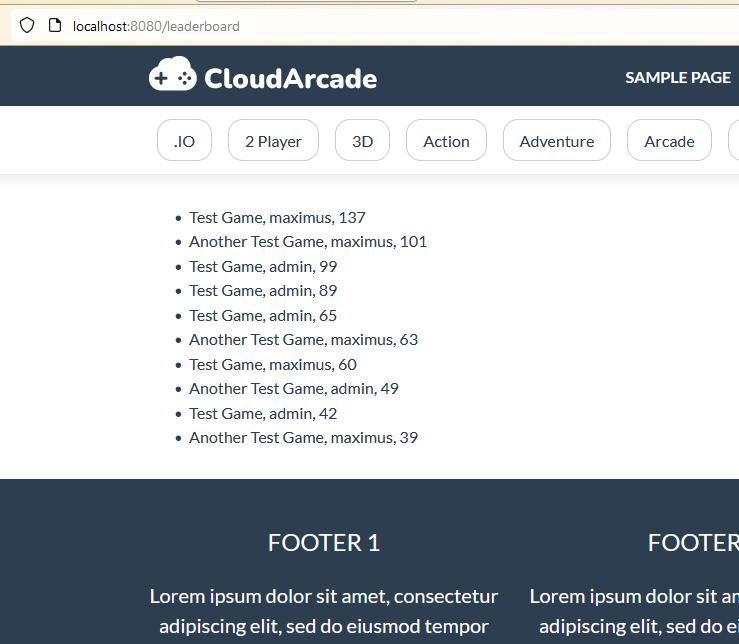
We fetch top 10 players from all games in the last 1 month
The looks is very basic, let’s implement bootstrap table.
<?php
$page_title = 'Leaderboard';
$meta_description = 'Top players';
require_once( TEMPLATE_PATH . '/functions.php' ); //Load theme functions
include TEMPLATE_PATH . "/includes/header.php";
// CONTENT
?>
<div class="container">
<div class="game-container">
<?php
$amount = 10;
$conn = open_connection();
$sql = "SELECT * FROM scores WHERE created_date > DATE_SUB(NOW(), INTERVAL 1 MONTH) ORDER by score DESC LIMIT ".$amount;
$st = $conn->prepare($sql);
$st->execute();
//
$row = $st->fetchAll(PDO::FETCH_ASSOC);
$list = [];
foreach($row as $item){
$game = Game::getById($item['game_id']);
if($game){
$item['game_title'] = $game->title;
$item['username'] = User::getById($item['user_id'])->username;
array_push($list, $item);
}
}
//Show the list
?>
<h3>Top Players</h3>
<br>
<table class="table table-bordered">
<thead>
<tr>
<th scope="col">#</th>
<th scope="col">Usename</th>
<th scope="col">Game Title</th>
<th scope="col">Score</th>
</tr>
</thead>
<tbody>
<?php
$index = 0;
foreach($list as $item){
$index++;
?>
<tr>
<th scope="row"><?php echo $index ?></th>
<td><?php echo $item['username'] ?></td>
<td><?php echo $item['game_title'] ?></td>
<td><?php echo $item['score'] ?></td>
</tr>
<?php
}
?>
</tbody>
</table>
</div>
</div>
<?php
include TEMPLATE_PATH . "/includes/footer.php"
?>
Here is the result:
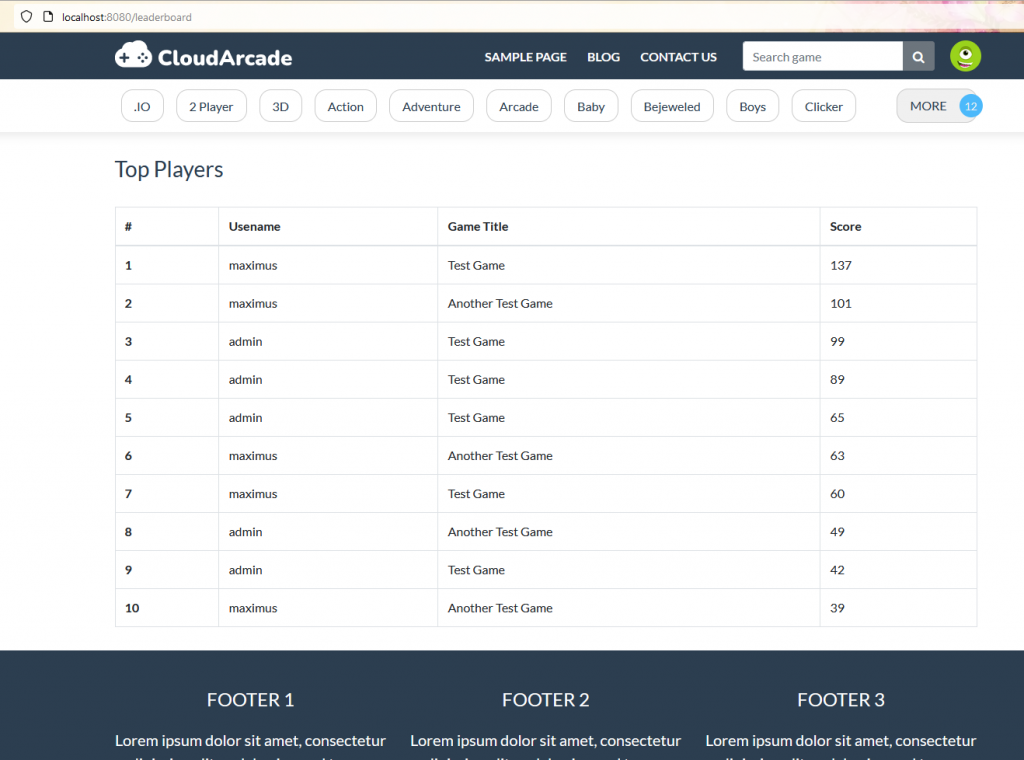
Look better right ?
You can explore the api script at “/includes/api.php”, also there are a JavaScript version Game API
If a player submitted a score, their previous score will not be removed, so there will be a duplicates, you can fix it by filtering the result.
You can put multiple leaderboard on a single page.