Customize page title
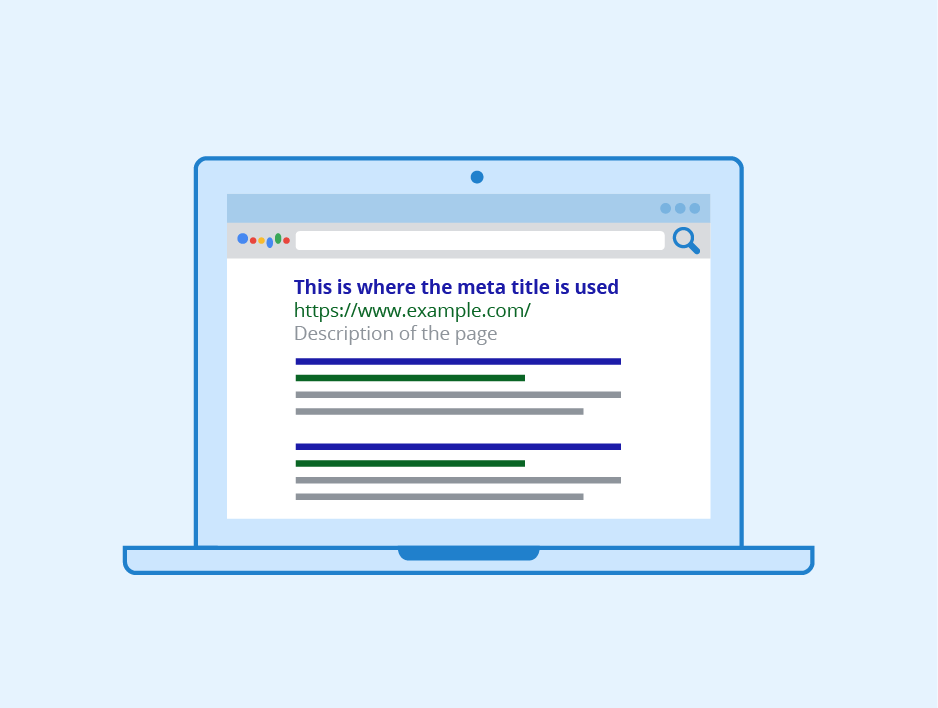
Starting from CloudArcade v1.6.5, you can either customize all page titles or customize a single content type, such as the game page title.
By default, you can retrieve the page title as follows:
<title><?php echo get_page_title() ?></title>
If you leave the function parameter empty, it will return a default title format that most likely will look like this: “CONTENT TITLE | SITE DESCRIPTION”.
How to Customize It?
To customize the page title, you’ll need to write custom PHP code. If you’re using the stock theme, you should place your custom PHP code in custom.php
so that your code is not overridden during theme updates. If you’re using a custom theme, you can place it in either functions.php
or custom.php
.
Here is a basic sample code to create a custom page title:
function get_custom_page_title(){
// Must return a string value
global $base_taxonomy;
$content_title = 'default';
switch($base_taxonomy){
case 'game':
global $game;
$content_title = 'GAME TITLE: '.$game->title.' * '.SITE_TITLE.' * '.SITE_DESCRIPTION;
break;
case 'category':
global $category;
$content_title = 'CATEGORY NAME: '.$category->name;
break;
default:
break;
}
return $content_title;
}
Alternatively, you can use an if-else statement:
function get_custom_page_title(){
// Must return a string value
global $base_taxonomy;
$content_title = 'default';
if($base_taxonomy == 'game'){
global $game;
$content_title = 'GAME TITLE: '.$game->title.' * '.SITE_TITLE.' * '.SITE_DESCRIPTION;
} elseif($base_taxonomy == 'category'){
global $category;
$content_title = 'CATEGORY NAME: '.$category->name;
}
return $content_title;
}
The function name must be get_custom_page_title()
.
With this code, if you visit a game page, the page title will be “GAME TITLE: Cooking Mania * Cloud Arcade * Play HTML5 Games”. If you visit a category page, the page title will be “CATEGORY NAME: Arcade”.
You can combine this with global functions and variables to suit your needs.
If another taxonomy is not set, the default preset “CONTENT TITLE | SITE DESCRIPTION” will be used.
The function get_custom_page_title()
must return a string value, and the default return value should be “default”.
If you want to explore other content types, you can copy the code from the get_page_title()
section in the file content/themes/theme-functions.php
.
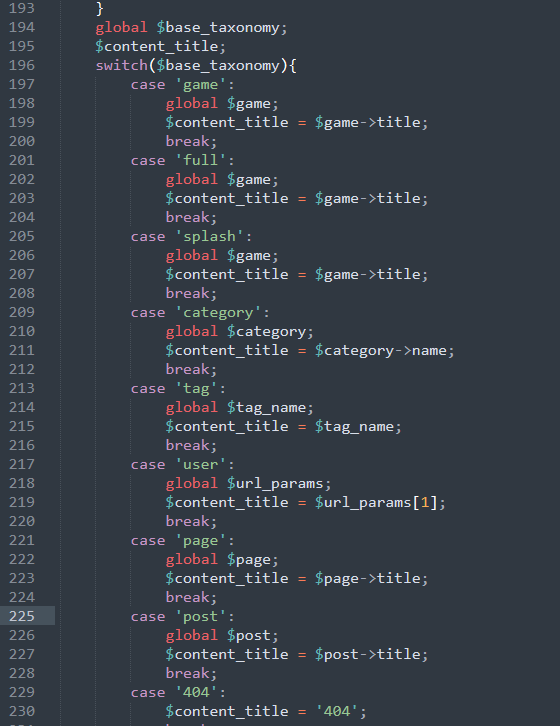